Five Must-Know TypeScript Features for Effective Coding
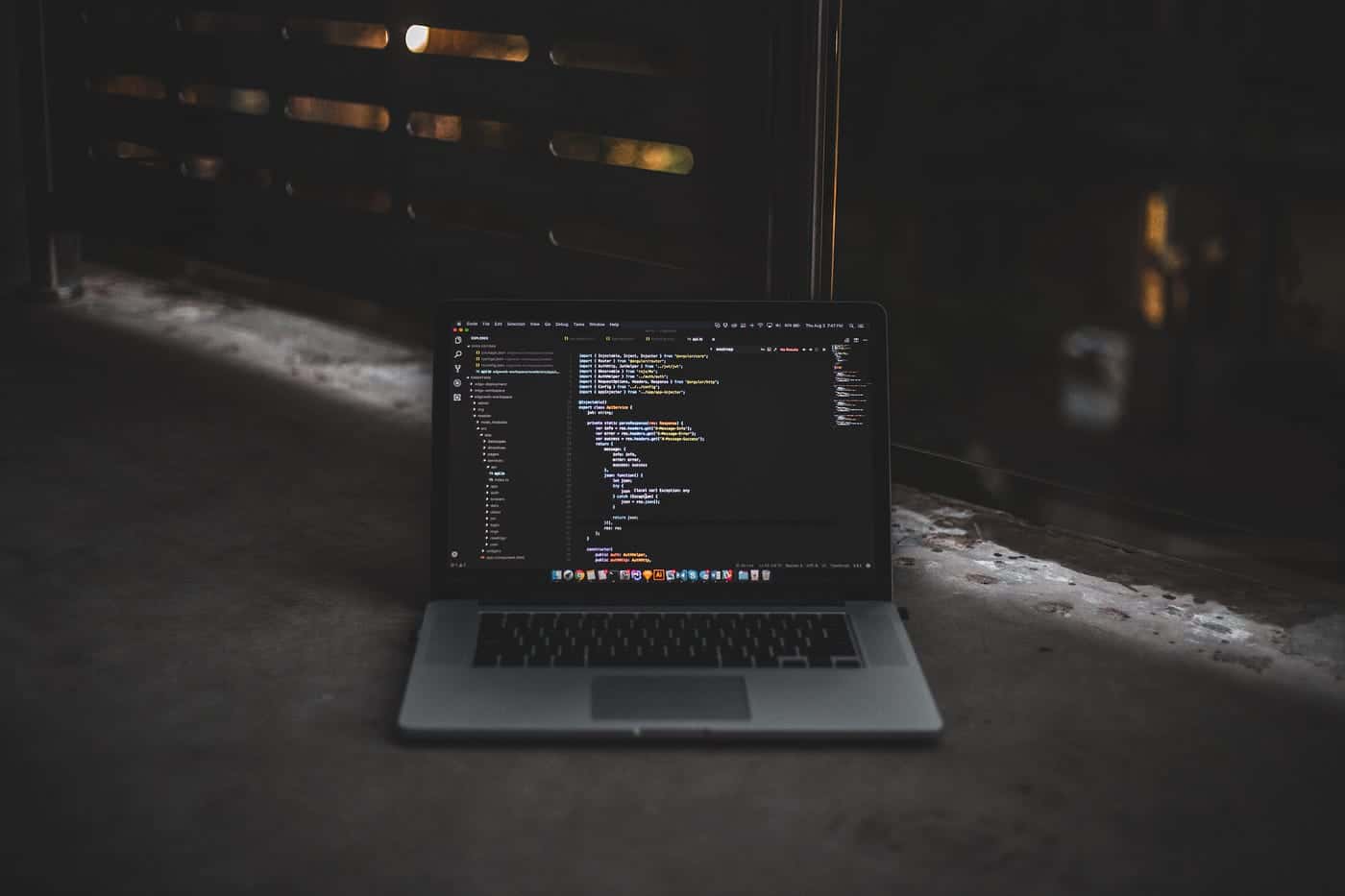
TypeScript has become an essential tool for many JavaScript developers, thanks to its ability to add strong typing and other features that optimize for scale and maintainability. In this guide, we walk through five essential TypeScript features that you should be using in your projects.
TypeScript's Interface System:
Interfaces in TypeScript serve to define the schema for objects, offering a way to enforce a certain shape and attribute set. This is highly valuable for avoiding bugs and improving code readability.
Example:
interface Individual {
name: string;
age: number;
greet(): void;
}
const person: Individual = {
name: 'Jane Doe',
age: 25,
greet(): void {
console.log(`Hi, I'm ${this.name}`);
}
};
The Power of Classes in TypeScript:
In TypeScript, classes are your go-to construct for object-oriented programming. With the support for inheritance and polymorphism, classes simplify code structure and make it easier to manage complex systems.
Example:
class Creature {
name: string;
constructor(name: string) {
this.name = name;
}
greet(): void {
console.log(`Hi, I'm ${this.name}`);
}
}
class Feline extends Creature {
species: string;
constructor(name: string, species: string) {
super(name);
this.species = species;
}
greet(): void {
console.log(`Hi, I'm ${this.name} and I'm a ${this.species}`);
}
}
Leverage Generics for Code Reusability:
TypeScript's generic types enable the creation of reusable components. This feature saves time and prevents errors by allowing developers to use a variety of types with a single function or class.
Example:
function listElements<T>(arr: T[]): void {
arr.forEach(item => console.log(item));
}
listElements<string>(['TypeScript', 'JavaScript']);
listElements<number>([10, 20, 30]);
Use Decorators for Meta-Programming:
TypeScript decorators allow you to annotate and modify classes and properties at design time. This enables more maintainable code by handling cross-cutting concerns like logging or validation.
Example:
function logExecution(target: any, methodName: string, descriptor: PropertyDescriptor) {
const originalFunction = descriptor.value;
descriptor.value = function(...args: any[]) {
console.log(`Executing ${methodName} with arguments: ${JSON.stringify(args)}`);
const output = originalFunction.apply(this, args);
console.log(`The method ${methodName} returned: ${JSON.stringify(output)}`);
return output;
};
return descriptor;
}
class MathOps {
@logExecution
sum(x: number, y: number): number {
return x + y;
}
}
const mathOps = new MathOps();
mathOps.sum(5, 7);
Organize Code with TypeScript Modules:
Modules in TypeScript aid in code separation and reusability. They offer an organized way to include various parts of your project without causing a tangle of dependencies.
Example:
// utility.ts
export const greeting = 'Hello, TypeScript!';
// app.ts
import { greeting } from './utility';
console.log(greeting);
Final Words
TypeScript elevates JavaScript development to a new level of professionalism and scalability. The features outlined above are instrumental in enhancing your TypeScript code quality. Whether you're a novice or a seasoned programmer, mastering these functionalities will not only improve your coding skills but will also lead to more robust applications.